Hướng dẫn upload file vào google drive sử dụng nodejs
1. Enable Google Drive API và tạo Credentials
Để liên kết giữa ứng dụng nodejs và google drive bạn cần tạo thông tin chứng thực truy xuất google drive trên google console
Bước 1: Truy cập vào link sau đó chọn Enable
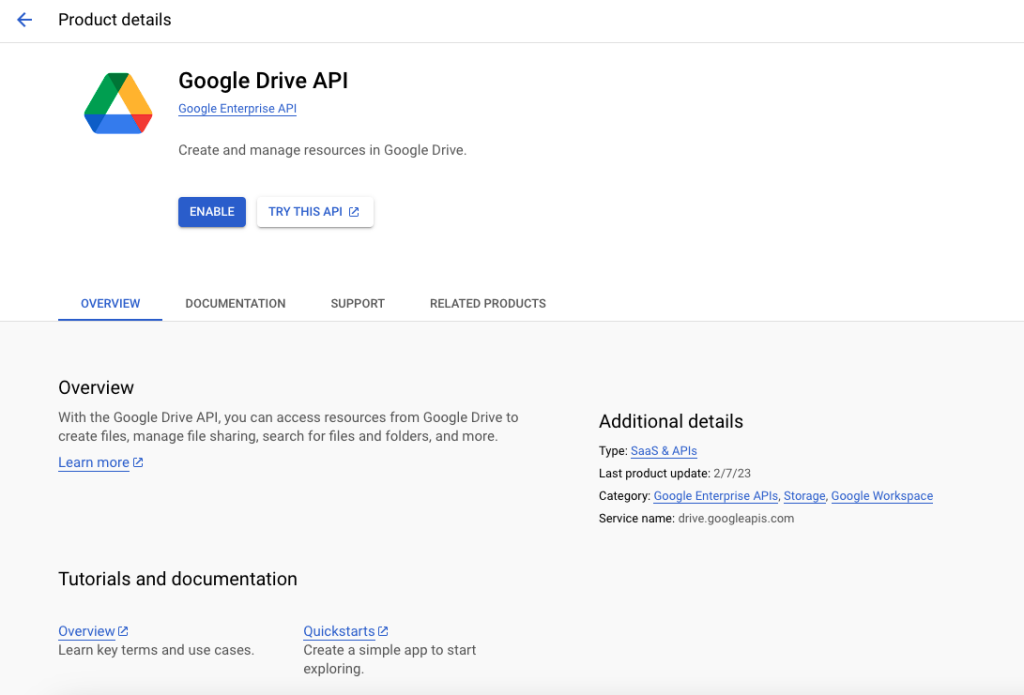
Bước 2: Sau khi Enable truy cập vào Manage, sau đó chọn tab Credentials > Service Account(như hình bên dưới)
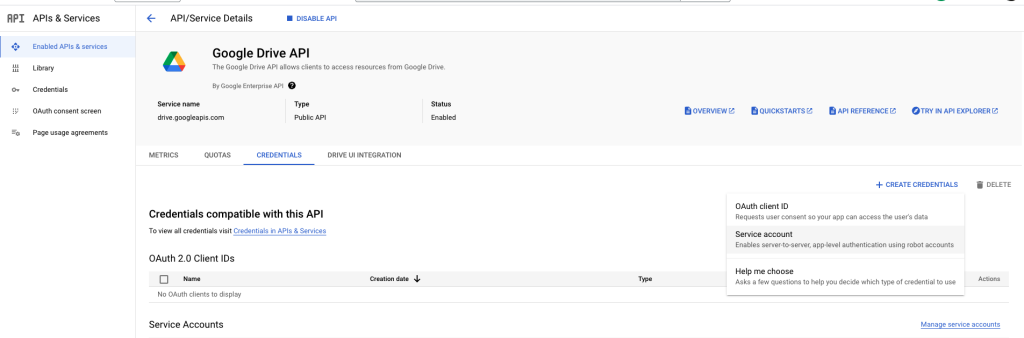
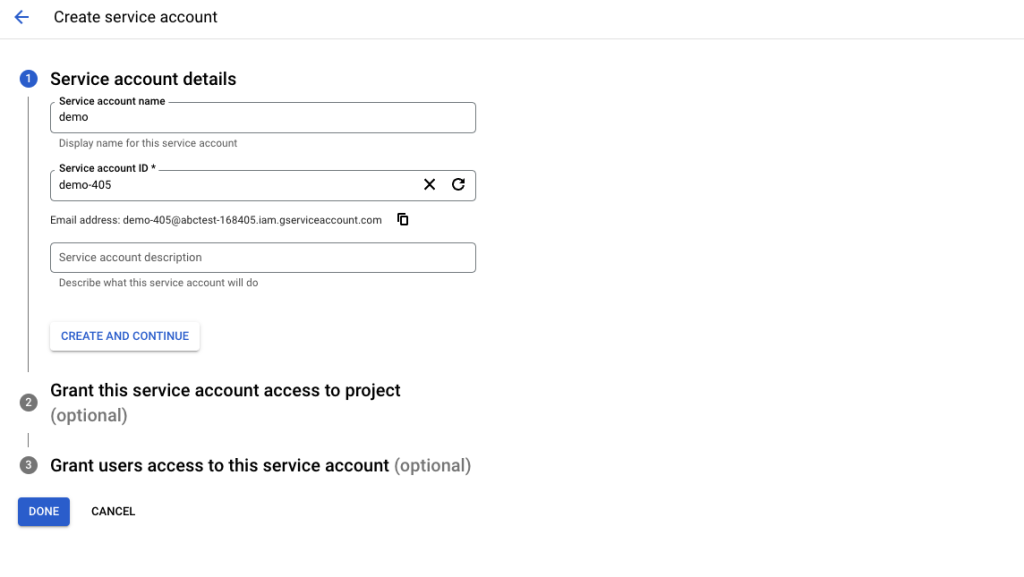
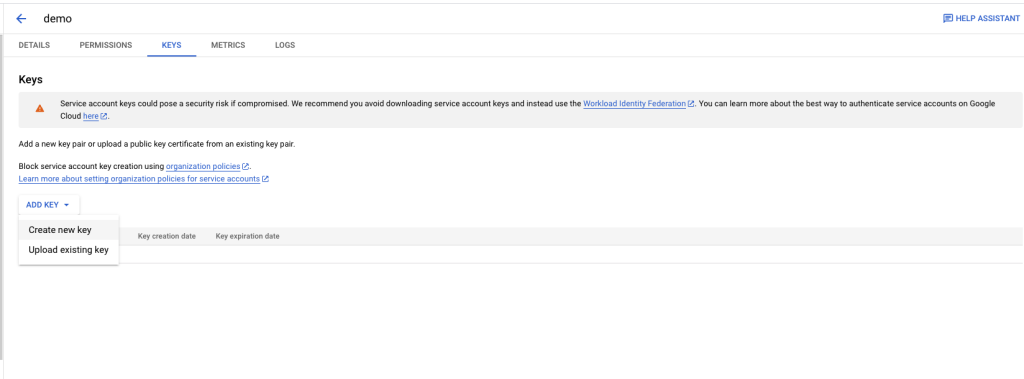
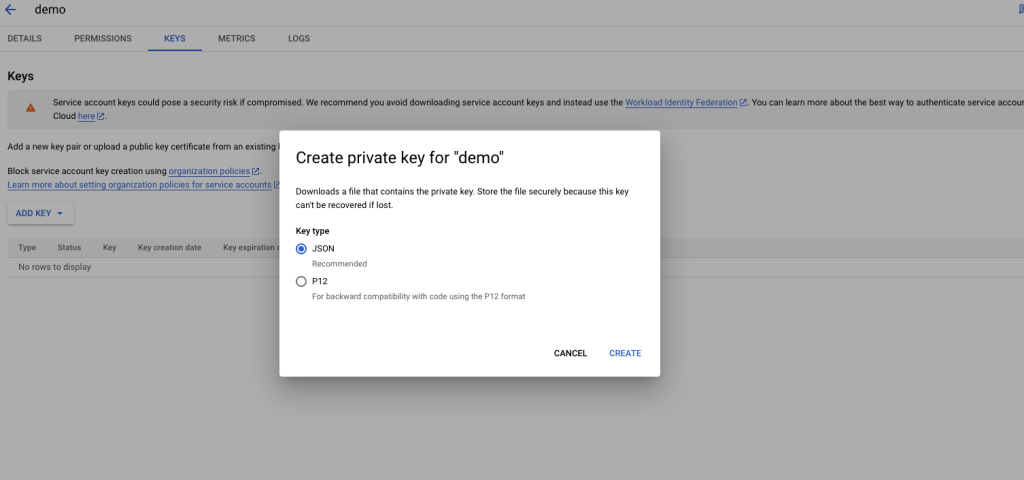
Key sẽ có định dạng như sau:
{
"type": "service_account",
"project_id": "your-project-id",
"private_key_id": "your-private-key-id",
"private_key": "your-private-key",
"client_email": "your-client-email",
"client_id": "your-client-id",
"auth_uri": "https://accounts.google.com/o/oauth2/auth",
"token_uri": "https://oauth2.googleapis.com/token",
"auth_provider_x509_cert_url": "https://www.googleapis.com/oauth2/v1/certs",
"client_x509_cert_url": "https://www.googleapis.com/robot/v1/metadata/x509/your-client-email"
}
2. Trên ứng dụng nodejs cài đặt package để handle xử lý upload và liên kết với ggdrive
npm install googleapis multer
3. Handle xử lý upload trong nodejs
const express = require('express');
const multer = require('multer');
const { google } = require('googleapis');
const app = express();
const upload = multer({ dest: 'uploads/' }); // Destination directory for storing uploaded files
// Configure Google Drive API credentials
const credentials = require('./path/to/credentials.json');
const auth = new google.auth.GoogleAuth({
credentials,
scopes: ['https://www.googleapis.com/auth/drive.file'],
});
// Handle file upload endpoint
app.post('/upload', upload.single('file'), async (req, res) => {
const { file } = req;
const drive = google.drive({ version: 'v3', auth });
const folderId = 'YOUR_FOLDER_ID';
try {
const response = await drive.files.create({
requestBody: {
name: file.originalname,
mimeType: file.mimetype,
parents: [folderId],
},
media: {
mimeType: file.mimetype,
body: require('fs').createReadStream(file.path),
},
});
res.status(200).json({ fileId: response.data.id });
} catch (error) {
console.error('Error uploading file:', error);
res.status(500).json({ error: 'Failed to upload file' });
}
});
// Start the server
app.listen(3000, () => {
console.log('Server is running on http://localhost:3000');
});
Trong đó:
- ./path/to/credentials.json: là đường dẫn đến file json vừa download ở bước 1
- YOUR_FOLDER_ID: là id của folder trên goodrive, ví dụ: https://drive.google.com/drive/folders/YOUR_FOLDER_ID
Ghi chú: Folder trên google drive bạn phải share quyền với client_email tương ứng trong file json download ở bước 1.
Chúc các bạn thành công!